I and my buddy William were working on a quick shopping cart project recently. We decided to implement Graphql as a new tech to handle our data query & manipulation. I spent some time this week trying to dip into Graphql as I have been using Express to create REST API for a long time and I heard a lot of hype about this tech.
As I know that REST API is created by the individual endpoint, whereas Graphql is focusing on the task itself. So, we can include all requests into one API which is a great way to reduce the workload.
I am using pieces of code from our shopping cart project to demonstrate the process of how we built up a basic Graphql query down below ( Not including the mutation part )
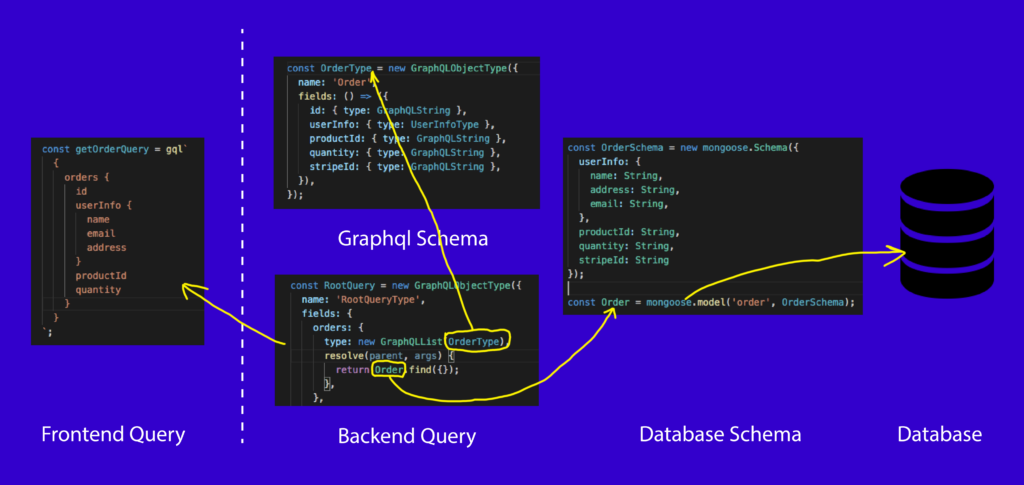
Here I got a general Graphql working flow and I am going to explain it from right to left.
1. Download packaging & build the graphql route
Let’s run the code below to install several packages.
Graphql : the main package we used to build our Graphql query
Express-graphql : the one we used to build our Graphql route
Cors : middleware for cross servers data transformation. ( between backend and frontend )
Apollo-boost : build Graphql client at the frontend
React-apollo : use its ‘apollo-provider’ to wrap around the frontend root file. So the child components could be able to access the Graphql result.
The code above is what the Graphql route is. We use the port we set at line 19, plus the ‘/graphql’ as the route path. In graphqlHTTP, we pass the Graphql schema which is another file we will build into the parameter.
2. Build the Graphql schema & its query
So, what those codes are saying is that we get our RootQuery which includes the action to print out all orders in the database at line 34. On line 35, we are using the Graphql schema from lines 11 to 20 as a reference to pull the data. In the resolve function, we will use the database schema that we imported on line 2 to find our answer in the database.
3. Setup the frontend client & query
Here we come to the setup of the frontend client which we use to connect to our backend route. You can see it from lines 4 to 6. Then we use the ApolloProvider tag to wrap our frontend root file in it. So many of our child components will be able to access the result coming back from Graphql.
In our child component, we need to set the query which includes the data format that we want to get from the result (lines 5 to 18 ). Don’t forget to bind the query name at the end of the export, so that we can access the returned data in our props. ( we console.log the props at line 22, and the result below ).
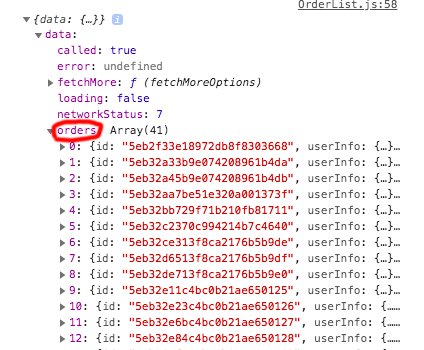