Higher Order Function
A higher order function is a function can take a function as an argument or return a function.
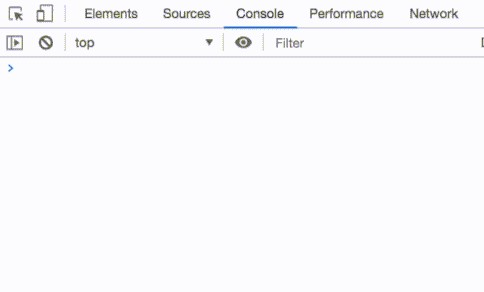
Closure?
A closure is the ability of a function to “remember” and “access” its lexical scope when it is executed outside of it. In short, a nested function’s inner function will have access to the value of the parent’s scope. But, make sure the inner function has to be physically placed in the parent function.
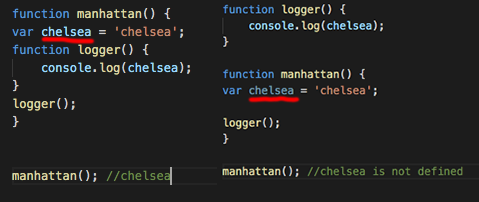
Hoisted
We all know that if you set a variable inside of a JS function, it will be hoisted to the top of the function as a way to be able to access its value anywhere within the function. But if you forget to assign you variable at line 3 in the picture below, the hoisted rule will elevate your variable outside of the function and put it at the top of your global scope( Terrible idea!! )
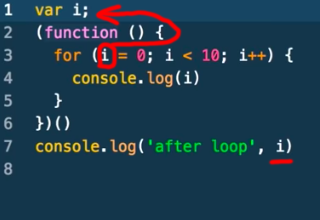
PS: That’s why people came up with the ‘use strict‘ to prevent it.
Var, Let & Const
Var : Because the less of locking ( Example above ), var can be hoisted accidentally (Stop using it ).
Let : Block scope, means it will lock the value inside of present scope.
Const : Can not be reassign value completely, but it can be changed partly.
PS: If we need to completely reassign a value to a variable, use Let, otherwise use Const
Should they bind?
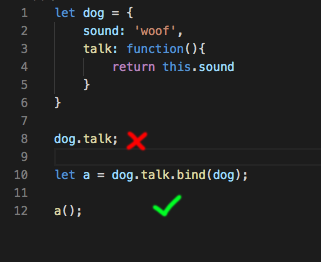
If you are assigning the function inside of the dog object outside its scope, you have to rebind it to the original scope.
Function Expression & Declaration
Declaration: Can be hoisted to the top of the code. Function expressions aren’t hoisted, which allows them to retain a copy of the local variables from the scope where they were defined ( Look up the sample in higher-order function ).
July 2, 2020
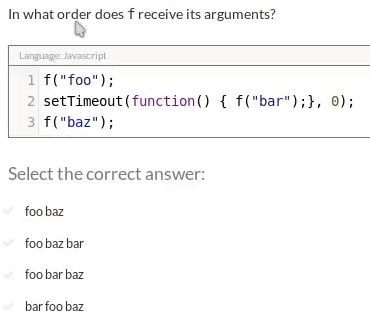
Whenever setTimeout runs. It always will let other codes to run first, even though the waiting period is 0. In the case of this question, we choose B.